Generate Stream URLs
With Dailymotion Pro, you have the freedom to deliver your content the way you want with the multiple solutions we offer!
To keep your content safely hosted at Dailymotion and integrate it with third-party players, you can generate unrestricted or secured stream URLs.
This guide will walk you through the process of generating stream URLs, from creating a private API key to delivering content.
Overview
- Create a Private API Key
- Generate stream URLs
- Deliver content to your end-users
1. Create a Private API Key
In order to authenticate against your Dailymotion account and retrieve an access token, you need to generate a private API Key that includes the required permission:
- Log in to your Dailymotion Studio
- Go to Organization Settings > API Keys > Create API Key > Private API Key
- Under Permissions > Manage videos, toggle on Generate Stream URLs
If you don’t see this permission, please reach out to your Dailymotion Account Manager - Click Create
- Copy and store your API key and secret in a secured location
For more details, check our full API Key guide.
2. Generate a stream URL
You have the possibility to choose between 2 types of stream URLs:
- a) Unrestricted stream URLs without time and IP restrictions
- b) Secured stream URLs with time and IP restrictions
This flexibility suits various needs and preferences and lets you choose the way you want to deliver your content.
a) Unrestricted stream URL
With unrestricted stream URLs, your content is accessible without any time or IP restrictions. This solution is best suited for static environments, shorter content or small embedded players requiring minimum loading time.
Use no_expire=1
and no_ip_lock=1
to generate an unrestricted stream URL:
// Replace <VIDEO_ID> with your own video ID
// Replace stream_hls_url with any supported video formats: https://developers.dailymotion.com/guides/generate-stream-urls/#list-of-supported-formats
curl -X GET -H 'Authorization: Bearer ${ACCESS_TOKEN}' \
'https://partner.api.dailymotion.com/rest/video/<VIDEO_ID>?fields=stream_hls_url&no_expire=1&no_ip_lock=1'
Code samples to generate unrestricted stream URLs
import requests
import os
# Please adapt CLIENT_ID and CLIENT_SECRET depending on your server architecture
CLIENT_ID = os.getenv("DAILYMOTION_CLIENT_ID")
CLIENT_SECRET = os.getenv("DAILYMOTION_CLIENT_SECRET")
DAILYMOTION_API_BASE_URL = "https://partner.api.dailymotion.com"
DAILYMOTION_API_OAUTH_TOKEN_URL = f"{DAILYMOTION_API_BASE_URL}/oauth/v1/token"
DAILYMOTION_API_VIDEO_URL = f"{DAILYMOTION_API_BASE_URL}/rest/video"
def fetch_dailymotion_api_access_token() -> str:
response = requests.post(
url=DAILYMOTION_API_OAUTH_TOKEN_URL,
data={
"grant_type": "client_credentials",
"client_id": CLIENT_ID,
"client_secret": CLIENT_SECRET,
},
timeout=2,
)
return response.json()["access_token"]
def fetch_stream_urls(
video_id: str,
video_formats: str,
):
response = requests.get(
url=f"{DAILYMOTION_API_VIDEO_URL}/{video_id}",
params={
"fields": video_formats,
"no_expire": 1,
"no_ip_lock": 1,
},
headers={
"Authorization": f"Bearer {fetch_dailymotion_api_access_token()}",
},
timeout=2,
)
return response.json()
<?php
# Please adapt CLIENT_ID and CLIENT_SECRET depending on your server architecture
define("CLIENT_ID", getenv("DAILYMOTION_CLIENT_ID"));
define("CLIENT_SECRET", getenv("DAILYMOTION_CLIENT_SECRET"));
const DAILYMOTION_API_BASE_URL = "https://partner.api.dailymotion.com";
const DAILYMOTION_API_OAUTH_TOKEN_URL = DAILYMOTION_API_BASE_URL . "/oauth/v1/token";
const DAILYMOTION_API_VIDEO_URL = DAILYMOTION_API_BASE_URL . "/rest/video";
function fetchDailymotionApiAccessToken(): string {
$ch = curl_init(DAILYMOTION_API_OAUTH_TOKEN_URL);
curl_setopt_array(
$ch,
[
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => [
"grant_type"=> "client_credentials",
"client_id" => CLIENT_ID,
"client_secret" => CLIENT_SECRET,
"scope" => "upload_videos,read_videos,edit_videos,delete_videos",
],
CURLOPT_TIMEOUT => 2,
CURLOPT_RETURNTRANSFER => true,
]
);
$response = curl_exec($ch);
curl_close($ch);
return json_decode($response, true)["access_token"];
}
function fetchStreamUrls(string $videoId, string $videoFormats, string $clientIp): array {
$ch = curl_init(
DAILYMOTION_API_VIDEO_URL . "/{$videoId}"
. "?fields={$videoFormats}"
. "&no_expire=1"
. "&no_ip_lock=1"
);
curl_setopt_array(
$ch,
[
CURLOPT_HTTPHEADER => ["authorization: Bearer " . fetchDailymotionApiAccessToken()],
CURLOPT_TIMEOUT => 2,
CURLOPT_RETURNTRANSFER => true,
]
);
$response = curl_exec($ch);
curl_close($ch);
return json_decode($response, true);
}
print_r(fetchStreamUrls());
b) Secured stream URL
Secured stream URLs are tied to the end-user’s IP address and only valid for a limited time. This ensures your content is shared in a secured way and cannot be accessed publicly or leaked.
// Replace <VIDEO_ID> with your own video ID
// Replace stream_hls_url with any supported video formats: https://developers.dailymotion.com/guides/generate-stream-urls/#list-of-supported-formats
// Replace <CLIENT_IP> with end-user s IP address (IPv4 only)
curl -X GET -H 'Authorization: Bearer ${ACCESS_TOKEN}' \
'https://partner.api.dailymotion.com/rest/video/<VIDEO_ID>?fields=stream_hls_url&client_ip=<CLIENT_IP>'
Set up a service to generate secured stream URLs
To ensure optimal security, we recommend setting up a backend service that will handle the generation of secured stream URLs for each of your clients.
Important: Each secured stream URL should be requested at the time of use and should not be stored or re-used for different viewers.
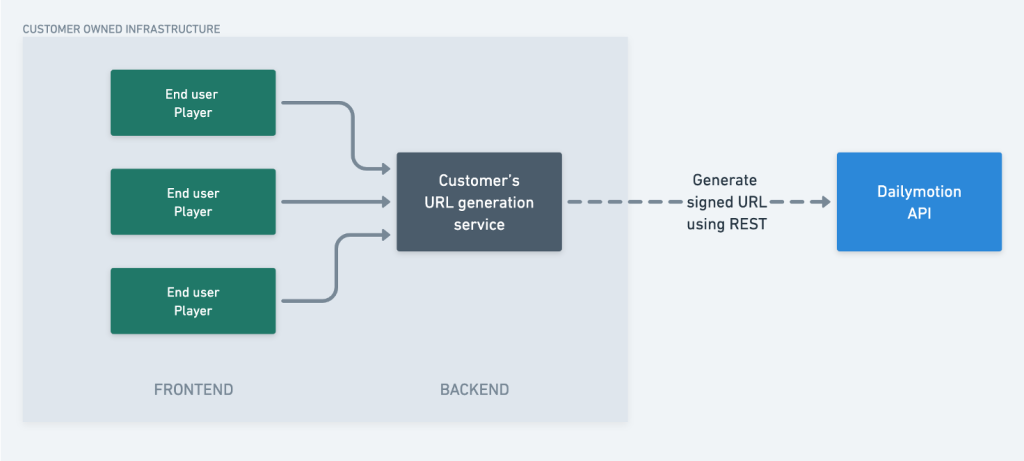
Sample service on GitHub
If you don’t have such service yet, we have put together a GitHub repository with basic building blocks to help you set up a sample service and generate secured stream URLs on demand.
Please note that this repository is meant to be a starting point: you will likely need to customize it to ensure that it meets all your security requirements and use cases before deploying it to production.
Code samples to generate secured stream URLs
If you already have a backend service of your own, the following code sample can be added within your server architecture to authenticate and generate secured stream URLs:
import requests
import os
# Please adapt CLIENT_ID and CLIENT_SECRET depending on your server architecture
CLIENT_ID = os.getenv("DAILYMOTION_CLIENT_ID")
CLIENT_SECRET = os.getenv("DAILYMOTION_CLIENT_SECRET")
DAILYMOTION_API_BASE_URL = "https://partner.api.dailymotion.com"
DAILYMOTION_API_OAUTH_TOKEN_URL = f"{DAILYMOTION_API_BASE_URL}/oauth/v1/token"
DAILYMOTION_API_VIDEO_URL = f"{DAILYMOTION_API_BASE_URL}/rest/video"
def fetch_dailymotion_api_access_token() -> str:
response = requests.post(
url=DAILYMOTION_API_OAUTH_TOKEN_URL,
data={
"grant_type": "client_credentials",
"client_id": CLIENT_ID,
"client_secret": CLIENT_SECRET,
},
timeout=2,
)
return response.json()["access_token"]
def fetch_stream_urls(
video_id: str,
video_formats: str,
client_ip: str,
):
response = requests.get(
url=f"{DAILYMOTION_API_VIDEO_URL}/{video_id}",
params={
"client_ip": client_ip,
"fields": video_formats,
},
headers={
"Authorization": f"Bearer {fetch_dailymotion_api_access_token()}",
},
timeout=2,
)
return response.json()
<?php
# Please adapt CLIENT_ID and CLIENT_SECRET depending on your server architecture
define("CLIENT_ID", getenv("DAILYMOTION_CLIENT_ID"));
define("CLIENT_SECRET", getenv("DAILYMOTION_CLIENT_SECRET"));
const DAILYMOTION_API_BASE_URL = "https://partner.api.dailymotion.com";
const DAILYMOTION_API_OAUTH_TOKEN_URL = DAILYMOTION_API_BASE_URL . "/oauth/v1/token";
const DAILYMOTION_API_VIDEO_URL = DAILYMOTION_API_BASE_URL . "/rest/video";
function fetchDailymotionApiAccessToken(): string {
$ch = curl_init(DAILYMOTION_API_OAUTH_TOKEN_URL);
curl_setopt_array(
$ch,
[
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => [
"grant_type"=> "client_credentials",
"client_id" => CLIENT_ID,
"client_secret" => CLIENT_SECRET,
"scope" => "upload_videos,read_videos,edit_videos,delete_videos",
],
CURLOPT_TIMEOUT => 2,
CURLOPT_RETURNTRANSFER => true,
]
);
$response = curl_exec($ch);
curl_close($ch);
return json_decode($response, true)["access_token"];
}
function fetchStreamUrls(string $videoId, string $videoFormats, string $clientIp): array {
$ch = curl_init(
DAILYMOTION_API_VIDEO_URL . "/{$videoId}"
. "?client_ip={$clientIp}"
. "&fields={$videoFormats}"
);
curl_setopt_array(
$ch,
[
CURLOPT_HTTPHEADER => ["authorization: Bearer " . fetchDailymotionApiAccessToken()],
CURLOPT_TIMEOUT => 2,
CURLOPT_RETURNTRANSFER => true,
]
);
$response = curl_exec($ch);
curl_close($ch);
return json_decode($response, true);
}
print_r(fetchStreamUrls());
Once the API request is finished, the server returns a JSON response containing the stream URL of the requested video.
3. Deliver content to end-users
Now that you retrieved the stream URL for the intended video, you can integrate it in your third-party player solution to provide your end-users with the requested content.
Example: see below how a stream URL can be added in an HTML5 player.
# Integrate the retrieved stream URL in a player
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="referrer" content="no-referrer">
<title>Demo</title>
</head>
<body>
<video-js id="demo" controls></video-js>
<link href="https://vjs.zencdn.net/7.21.1/video-js.css" rel="stylesheet" />
<script src="https://vjs.zencdn.net/7.21.1/video.min.js"></script>
<script type="text/javascript">
const apiUrl = '/stream-urls'
const videoPlayer = videojs('demo')
fetch(`${apiUrl}?video_id=<your_video_xid_here>&video_formats=stream_h264_url`)
.then((res) => res.json())
.then((data) => {
videoPlayer.src(data.stream_h264_url)
})
</script>
</body>
</html>
Supported formats
You can generate stream URLs in any of our supported video formats by passing the desired API parameter(s) in fields
.
If the selected format returns null
, this indicates that the requested format is not available for the specified video.
HLS manifests
Manifest | Quality | API parameter | Resolution | W x H |
HLS (m3u8) | Dynamically adjusted | stream_hls_url | Dynamically adjusted | Dynamically adjusted |
Live HLS (m3u8) | Dynamically adjusted | stream_live_hls_url | Dynamically adjusted | Dynamically adjusted |
MP4 formats
Format | Quality | API parameter | Resolution | W x H |
H264 (mp4) | – | stream_h264_url | 380p | 512 x 384 |
H264 (mp4) | hq | stream_h264_hq_url | 480p | 720 x 480 |
H264 (mp4) | hd | stream_h264_hd_url | 720p | 1280 x 720 |
H264 (mp4) | hd1080 | stream_h264_hd1080_url | 1080p | 1920 x 1080 |
H264 (mp4) | qhd | stream_h264_qhd_url | 1440p | 2560 x 1440 |
H264 (mp4) | uhd | stream_h264_uhd_url | 2160p | 3840 x 2160 |
Postman Collection
You can use the API call to generate stream URLs in a more graphical user interface via our ready-to-use Postman Collection. It includes the API requests filled with the endpoint and parameters to authenticate and generate an unrestricted or a secured stream URL.
All you need to do is filling the variable fields with your credentials and parameters values!
Download files from Dailymotion
If you need to download a file from a stream URL, follow these steps:
- Generate a H264 stream URL in the desired format (following steps 1 & 2 above)
- As shown in the example below, append the retrieved stream URL with
download=1
parameter to enable file download - Download the file using the link
# BASH example
wget https://www.dailymotion.com/cdn/H264-512x384/video/x730nnd.mp4?sec=4dXW1UzVoHWu7YyRlEXvXn_WQDTROD8sPgKTcnUems38Mmhktq1YlZF4jT4GshGm&download=1