Generate reports using a private API key
In this guide, we’ll show you the steps to authenticate and generate reports via the Reporting API using a private API key.
If you’re using a public API key instead, please follow the dedicated guide since endpoints used by public or private API keys aren’t the same.
1. Generate a private API key
Follow the dedicated guide to easily create a private API Key from your Dailymotion Studio.
While creating your private API key, make sure to toggle on any permissions you may need in the “Manage reports” section:
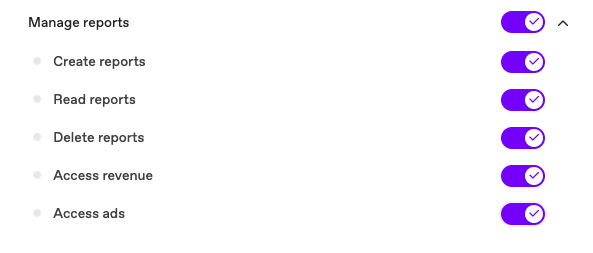
Once created, you’ll retrieve an API key and API secret. For security reasons, the API secret is only displayed once: make sure to store it safely before closing the window.
Move on to step 2 once this is done.
2. Perform authentication
Once you have your private API key, you can use it to generate an access token and authenticate.
Make a POST
request to the access token server https://partner.api.dailymotion.com/oauth/v1/token
with the following parameters:
grant_type
: set toclient_credentials
to specify the grant type flowclient_id
: the API key from step 1client_secret
: the API secret from step 1scope
: define specific scopes needed
Request example:
#!/usr/bin/env python
import requests
#Declaring authentication url
auth_url = "https://partner.api.dailymotion.com/oauth/v1/token"
#You can use your client_id and your client_secret generated to retrieve an access token
def get_access_token(client_id, client_secret):
'''
Authenticate on the API in order to get an access token
'''
response = requests.post(auth_url,
data={
'client_id': client_id,
'client_secret': client_secret,
'grant_type': 'client_credentials',
'scope': 'access_ads access_revenue create_reports delete_reports'
},
headers={
'Content-Type': 'application/json'
})
if response.status_code != 200 or not 'access_token' in response.json():
raise Exception('Invalid authentication response')
return response.json()['access_token']
#Calling the get_access_token function to retrieve an access token
access_token = get_access_token(<your_client_id>, <your_client_secret>)
#Creating an authorization header to use it in the report generation call request
authorization_header = {'Authorization': 'Bearer ' + access_token}
You have now retrieved an access token and have been authorized to access your reporting data via the API. Move on to step 3 to see how to generate a report.
3. Generate report
Now that you have your access token, you are ready to generate a report! Include the following elements in your API request:
- Endpoint: make a
POST
call tohttps://partner.api.dailymotion.com/graphql
for all subsequent API calls - Access token: passed in the request header via the
authorization_header
variable - Query: pass in the dimensions, metrics, filters, product and date range needed in the
create_report
function. Check the full Reporting API Reference to see the available parameters.
Request example:
# Report generation endpoint
generate_report_url = 'https://partner.api.dailymotion.com/graphql'
# Passing report parameters to the request. You can find the full list of parameters and their compatibility matrix here: https://developers.dailymotion.com/api/reporting-api/reference/
def create_report(metrics, dimensions, start_date, end_date, product, filters):
'''
Creating a report request
'''
reportRequest = """
mutation ($input: AskPartnerReportFileInput!) {
askPartnerReportFile(input: $input) {
reportFile {
reportToken
}
}
}
"""
response = requests.post(
generate_report_url,
json={
'query': reportRequest,
'variables': {
'input': {
'metrics': metrics,
'dimensions': dimensions,
'filters': filters,
'startDate': start_date,
'endDate': end_date,
'product': product,
}
}
},
headers=authorization_header
)
if response.status_code != 200 or not 'data' in response.json():
raise Exception('Invalid response')
return response.json()['data']['askPartnerReportFile']['reportFile']['reportToken']
# Calling the report generation function to generate a report. To see the full list of metrics, dimensions and filters, please visit this page: https://developers.dailymotion.com/api/reporting-api/reference/
report_token = create_report(["VIEWS"], ["DAY", "VIDEO_TITLE"], "2023-07-01", "2023-07-04", "CONTENT", {
"videoOwnerChannelSlug": "<your_channel_slug>"
})
In this example, we are generating a report gathering the VIEWS
per DAY
and VIDEO_TITLE
for the Dailymotion channel defined in the filter videoOwnerChannelSlug
. This report will only show data from the channel’s uploaded CONTENT
for the time frame 2023-07-01
to 2023-07-04
.
This request will generate a reportToken
that will be used to access the report once ready.
4. Download the report
Your report is generated and available once it displays the status FINISHED
. Note that it can take up to two hours for a report to be generated.
All you have to do now is to get the report link and download your report.
#The report status endpoint is the same used for report creation in step 3. For simplicity the authentication step was omitted in this example
report_status_url = 'https://partner.api.dailymotion.com/graphql'
def check_report_status(report_token):
'''
Checking the status of the reporting request
'''
report_request_status_check = """
query PartnerGetReportFile ($reportToken: String!) {
partner {
reportFile(reportToken: $reportToken) {
status
downloadLinks {
edges {
node {
link
}
}
}
}
}
}
"""
response = requests.post(
report_status_url,
json={
'query': report_request_status_check,
'variables': {
'reportToken': report_token
}
},
headers= authorization_header
)
if response.status_code != 200 or not 'data' in response.json():
raise Exception('Invalid response')
status = response.json()['data']['partner']['reportFile']['status'];
if (status == 'FINISHED'):
download_links = []
for url in map(lambda edge: edge['node']['link'], response.json()['data']['partner']['reportFile']['downloadLinks']['edges']):
download_links.append(url)
return download_links
else:
return None
#Getting the download url of the report when the status is equals to 'FINISHED'report_download_url = check_report_status(report_token)